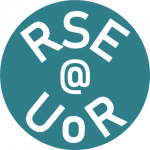
Glossary of terms
Colours
Below is a summary of the predefined colours from the color object and their associated vectors.
color.red: vector(1,0,0)
color.yellow: vector(1,1,0)
color.black: vector(0,0,0)
color.green: vector (0,1,0)
color.orange: vector(1,0.6,0)
color.white: vector(1,1,1)
color.blue: vector(0,0,1)
color.cyan: vector(0,1,1)
color.purple: vector(0.4,0.2,0.6)
color.magenta: vector(1,0,1)
A visual guide to colors inVPython can be found here: VPython Color-RGB-HSV
Vectors
Not all objects in VPython are visible objects. For example colour and sizes.
Individual parts of a vector can be accessed using the dot notation, for example the z component of a myvector(1,2,3) can be accessed by calling myvector.z which will return 3.
VPython allows you to perform vector operations on them. You do not need to add the components one by one; VPython will do the vector addition for you...
Figure 1 shows the graphical representation of two vectors and how the two vectors add together to produce a resultant.
Use the mouse to rotate the figure around to see the effect.
a = vector(2,3,-4) # can also be written briefly as vec(2,3,-4)
b = vector(4,-1,8)
c = a+b
print(c)
If you include print(c), you will see that it is a vector with components < 6, 2, 4 >.
Scalar multiplication
d = 3*a # d is a vector with components <12, -3, 24>
Vector magnitude
s = mag(c) # s is a scalar magnitude [ 7.48331 ]
z = mag(c)**2 # square the magnitude of the vector; ** means "to the power of"
Vector products
f = cross(a,b) # cross product [ < -20, 32, 14 >]
g = dot(a,b) # dot product [-27 ]
h = norm(a) # normalized (unit) vector; a/mag(a); same as hat(a) or a.hat [ < 0.444444, -0.111111, 0.888889 >]
The attributes of VPython objects can be vectors, such as velocity or momentum.
Simple Python Programming
Comments
A comment in a Python program starts with "#"
# This line is a comment
'''This line is a
multiline comment
'''
Variables
Variables can be created anywhere in a Python program, simply by assigning a variable name to a value.
The type of the variable is determined by the assignment statement.
a = 3 # an integer
b = -2.4 # a floating-point number
c = vector(0.4, 3e3, -1e1) # a vector
Earth = sphere(pos=vector(0,0,0), radius=6.4e6) # a 3D object
bodies = [ship, Earth, Moon] # a list of objects
mycar={'make':'Nissan','model':'Micra','engine':1.2} # a dictionary
Basic VPython objects such as sphere(), box() have a set of "attributes" such as color.
User defined objects such as Earth=sphere() can have user defined attribute such as Earth.momentum=34
Exponentiation
** represets 'to the power of' eg x squared would be x**2, xcubed would be x**3
Logical Tests
Logical tests like if...else or loops like for x in y and while True< must end in a colon and the next line is indented
if a == b: # note the double equal sign for "is equal"
c = 5 # executed if a is equal to b
d = 5*a # also executed if a is equal to b
elif a > 10: # a not equal to b but greater than 10
x = a+b
else: # any other case
x = b/a
Note the obligatory indentation for the action after the if statement
Logical expressions
== |
equal |
!= |
not equal |
< |
less than |
> |
greater than |
<= |
less than or equal |
>= |
greater or equal |
or |
logical or |
and |
logical and |
in |
member of a sequence |
not in |
not sequence member |
Lists
A list is an ordered sequence of any kind of object. It is delimited by square[] brackets.
moons = [Io, Europa, Ganymede, Callisto]
Lists in python are arrays and indexed from zero
For example, Ganymede in the above list has index 2 and can be accessed using the notation moons[2]
The function "arange" (short for "arrayrange") creates an array of numbers:
angles = arange (0, 2*pi, pi/100)
# from 0 to 2*pi-(pi/100) in steps of (pi/100)
numbers = arange(5) # the arange function creates a list of numbers from 0 to n
for i in numbers:
print(i) # prints 0, 1, 2, 3, 4
Another way to use arange is to give it arguments in the form arange(start, end, step)
The final range will always be 'end -1', so if you want the range 10 to 50 in steps of ten for example you will need to specify arange(10,60,10)
If step is missed out in the above then the array will be 10 to 59 in steps of 1
numbers = arange(10,60,10)
for i in numbers:
print(i) # prints 10, 20, 30, 40, 50
note the indentation or an error will result
Dictionaries
A dictionary is an ordered sequence of any kind of object, in { key:value pairs }
. It is delimited by curly{} brackets.
mycars={'mycar':'Nissan','dream_car':'Bugatti'} # a dictionary
To access a value refer to the key like this: x=mycars['dream_car']. x will equal 'Bugatti'
for key in mycars:
value=mycars[key]
print(key+':'+value+'\n')
note the indentation or an error will result
prints
'mycar':Nissan
dream_car:Bugatti
Loops
The simplest loop in Python is a "while" loop. The loop continues as long as the specified logical expression is true (note the obligatory indentation):
x=1
while x < 23:
print(x)
x += 1
Prints values 1-22.
If you want to get values 1-23 then change the while clause to while x<=23
note the indentation or an error will result
To write an infinite loop, just use a logical expression that will always be true:
while True:
rate(30) # limit animation rate, render scene at 30 frames per second (fps)
ball.pos = ball.pos + (ball.momentum/ball.mass)*dt
Accidental infinite loops are ok, because you can always interrupt the program by clicking "Edit this program".
However, you MUST include a rate statement in the animation loop.
It is also possible to loop over the members of a sequence:
moons = [Io, Europa, Ganymede, Callisto]
for a in moons:
r = a.pos - Jupiter.pos
for x in arange(10):
# see "lists" above
...
for theta in arange(0., 2.*pi, pi/100.):
# see "lists" above
if a == b: continue # go back to the start of the loop
if a > b: break # exit the loop
Exercise 1
Use the for..in: loop to print out a list of 5 planets: planets=['Mercury','Venus','Earth','Mars','Jupiter', 'Saturn']
and the if statement to append " my favourite!" to the output in a print statement if the planet is Earth
planets=['Mercury','Venus','Earth','Mars','Jupiter', 'Saturn']Solution to Exercise 1
string_to_print="My planets: \n"
for p in planets:
if p=='Earth':
string_to_print += p + " my favourite!\n" # notice the +=. This is shorthand for 'add and return result'
else:
string_to_print+=p+"\n"
print(string_to_print)
Exercise 2
Use the for..in: loop to print out a list of 5 planets from the dictionary {'planet 0':'Mercury','planet 1':'Venus','planet 2':'Earth','planet 3':'Mars','planet 4':'Jupiter','planet 5':'Saturn'}
and the if statement to append " my favourite!" to the output in a print statement if the planet is Earth as in exercise 1
planets={'planet 1':'Mercury','planet 0':'Venus','planet 2':'Earth','planet 3':'Mars','planet 4':'Jupiter', 'Saturn'}Solution to Exercise 2
string_to_print="My planets: \n"
for key in planets:
if key=='planet 2':
string_to_print += planets[key] + " my favourite!\n"
else:
string_to_print+=key +" " + planets[key]+"\n"
print(string_to_print)