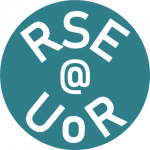
Lesson 11 - Setting the Scene
The scene object controls the view of the 3-D world and controls the lighting of it.
Here we look at the some of the commonly used settings which set the initial conditions when the user runs the program.
Timings
Teaching: 40 min
Exercises: 20 min
Objectives
By the end of the lesson the student should be able to:
Set up a scene to display a program with a customised title and caption.
Set up a scene to control the initial view the user sees of the program objects.
Scene properties
scene.width Sets the width of the scene window in pixels.
scene.height Sets the height of the scene window in pixels.
scene.title Sets the scene title above the ouput window.
scene.caption Sets caption text below the output window.
scene.range Sets the viewing width of the scene window in relative units.
scene.center Sets where the center of the scene is (normally the middle).
scene.forward Sets the angle at which the camera is pointing (default is minus Z), into screen.
scene.userspin Sets whether the user can use mouse keys to rotate the scene (True or False).
scene.userzoom Sets whether the user can use mouse keys to zoom in and out (True or False).
scene.background Sets background color as a shade of grey: zero=black, 1=white.
scene.ambient Sets ambient lighting strength: zero=dark, 1=bright.
scene.fov Sets the 'field of view' that the camera can see. It is an angle in radians. Small angles give a 2-D effect.
There are many more options for controlling the scene, which can be found at https://www.glowscript.org/docs/VPythonDocs/canvas.html.
The program
The code illustrates the various options that allow the user to control the scene.
scene.width = 800
scene.height = 800
scene.resizable = True
scene.range = 3
scene.fov = pi/2 #angle in radians. Small angles give a 2-D effect.
scene.center = vector(0,0,0) # a vector to center of screen
scene.forward = vector(1,1,1)
scene.userspin = True
scene.userzoom = True
scene.background = color.gray(0.9)
scene.ambient = color.gray(0.7)
scene.title="Exercise 1 - Setting the Scene"
scene.caption = """
Figure 1 - Viewing at an angle
"""
center=vector(0,0,0)
b = box(pos=center, size=vector(2,2,2), color=color.yellow)
v=arrow(pos=vec(0,2.2,-2),axis=scene.forward,length=3, shaftwidth=0.2, color=color.red, round=True)
Things to note
Background and ambient light are limited to shades of grey and the function color.gray() must be used to set it.
In figure 1 an arrow is added to help 'see' the viewing angle.
Exercise 1
Copy the program above and change the values of background, ambient, range, userspin, userzoom and range
to get a feel for what these settings do.
Conclusion
You should now be able to:
Understand more about the 3-D space inside the scene and change the scene settings to give a desired effect.
Modify the output window to present a program with a title and some information about the program in the caption.